Digital elevation models¶
Procedures for the following global products are currently implemented:
- SRTM 1 arcsecond
- SRTM 3 arcsecond
SRTM 1 arc second¶
Note
SRTM 1 arc second data is obtained from http://step.esa.int/auxdata/dem/SRTMGL1/
Create an object¶
from warsa.dem.srtm import SRTM1ARCSEC
srtm = SRTM1ARCSEC()
Create shapefile from tiles¶
The resulting shapefile has the file name of the corresponding tile as attribute.
srtm.create_layers()

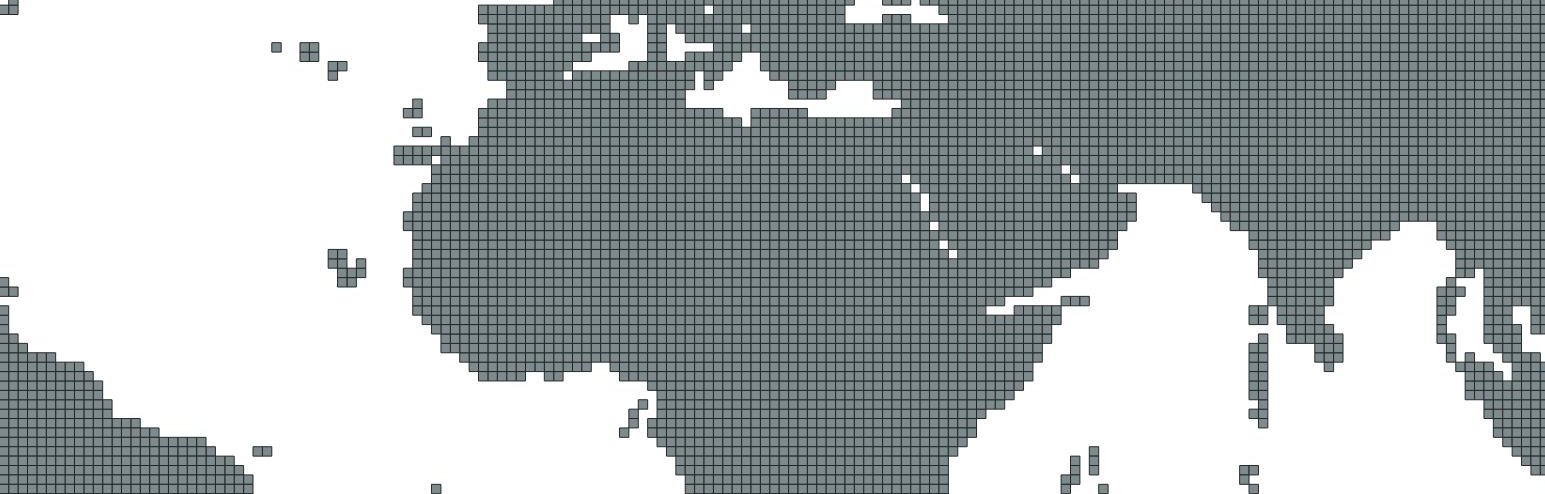
Create a merged dem from shapefile¶
This procedure merges (mosaics) all SRTM 1 arcsecond tiles intersected by the given shapefile. They will also be downloaded if necessary. Example:
srtm.merge_by_layers('/media/win/PythonProjects/warsadev/data/BuziWatershedWGS84.shp',
output_raster='/media/win/tmp/srtm_buzi.tif')
which is equivalent to:
tiles = srtm.get_tile_names_by_layers('/media/win/PythonProjects/warsadev/data/BuziWatershedWGS84.shp')
srtm.merge(tiles, output_raster='/media/win/tmp/srtm_buzi.tif')
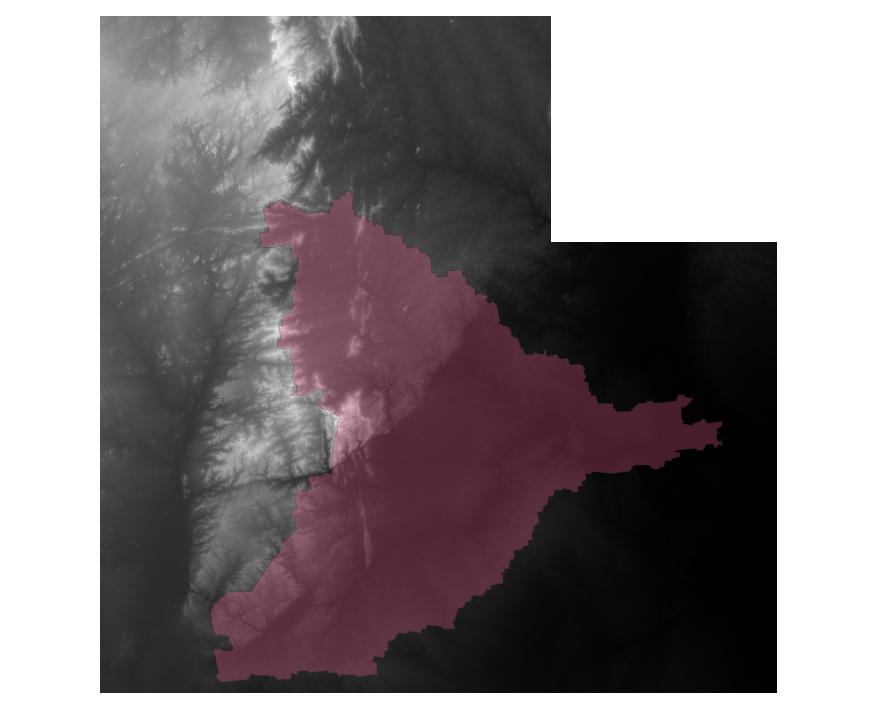
Download tiles¶
Tiles are downloaded in a directory srtm_1arcsec
, which is saved in the directory defined in the configuration file under dem
(see Configuration).
Downloading all tiles (it takes long):
srtm.download()
Output:
Downloading 10014 SRTM 1 arc files
1: downloading N00E009.SRTMGL1.hgt.zip... done in 14.68 seconds
2: downloading N00E011.SRTMGL1.hgt.zip... done in 24.55 seconds
3: downloading N00E012.SRTMGL1.hgt.zip... done in 39.16 seconds
4: downloading N00E013.SRTMGL1.hgt.zip... done in 27.94 seconds
5: downloading N00E014.SRTMGL1.hgt.zip... done in 50.40 seconds
6: downloading N00E016.SRTMGL1.hgt.zip... done in 65.58 seconds
7: downloading N00E017.SRTMGL1.hgt.zip... done in 3.61 seconds
8: downloading N00E018.SRTMGL1.hgt.zip... done in 1.77 seconds
9: downloading N00E019.SRTMGL1.hgt.zip... done in 1.72 seconds
10: downloading N00E021.SRTMGL1.hgt.zip... done in 1.82 seconds
11: downloading N00E022.SRTMGL1.hgt.zip...
Downloading tiles intersecting a shapefile:
srtm.download_by_layers('/media/win/PythonProjects/warsadev/data/BuziWatershedWGS84.shp')
Downloading tiles by name:
srtm.download_by_tile_names(['S07W065.SRTMGL1.hgt.zip', 'S07W066.SRTMGL1.hgt.zip',
'S08W065.SRTMGL1.hgt.zip', 'S08W066.SRTMGL1.hgt.zip'])
Output:
Downloading 4 SRTM 1 arc files
1: downloading S07W065.SRTMGL1.hgt.zip... done in 29.88 seconds
2: downloading S07W066.SRTMGL1.hgt.zip... done in 5.57 seconds
3: downloading S08W065.SRTMGL1.hgt.zip... done in 16.84 seconds
4: downloading S08W066.SRTMGL1.hgt.zip... done in 26.90 seconds
Get tile names¶
print(srtm.get_tile_name_by_point(-67.5, -6.5))
print(srtm.get_tile_names_by_extent(-67.5, -64.5, -6.5, -6.3))
print(srtm.get_tile_names_by_layers('/media/win/PythonProjects/warsadev/data BuziWatershedWGS84.shp'))
Output:
/media/roehrig/SARP/dem/srtm_1arcsec/S07W068.SRTMGL1.hgt.zip
['/media/roehrig/SARP/dem/srtm_1arcsec/S07W065.SRTMGL1.hgt.zip', '/media/roehrig/SARP/dem/srtm_1arcsec/S07W066.SRTMGL1.hgt.zip', '/media/roehrig/SARP/dem/srtm_1arcsec/S07W067.SRTMGL1.hgt.zip', '/media/roehrig/SARP/dem/srtm_1arcsec/S07W068.SRTMGL1.hgt.zip']
['/media/roehrig/SARP/dem/srtm_1arcsec/S19E032.SRTMGL1.hgt.zip', '/media/roehrig/SARP/dem/srtm_1arcsec/S19E033.SRTMGL1.hgt.zip', '/media/roehrig/SARP/dem/srtm_1arcsec/S20E032.SRTMGL1.hgt.zip', '/media/roehrig/SARP/dem/srtm_1arcsec/S20E033.SRTMGL1.hgt.zip', '/media/roehrig/SARP/dem/srtm_1arcsec/S20E034.SRTMGL1.hgt.zip', '/media/roehrig/SARP/dem/srtm_1arcsec/S21E032.SRTMGL1.hgt.zip', '/media/roehrig/SARP/dem/srtm_1arcsec/S21E033.SRTMGL1.hgt.zip', '/media/roehrig/SARP/dem/srtm_1arcsec/S21E034.SRTMGL1.hgt.zip']
SRTM 3 arc seconds¶
Note
SRTM 3 arc second data is obtained from http://srtm.csi.cgiar.org
In each downloaded srtm3 zip-file you will find, among others:
DISTRIBUTION
Users are prohibited from any commercial, non-free resale, or redistribution without explicit written permission from CIAT. Users should acknowledge CIAT as the source used in the creation of any reports, publications, new data sets, derived products, or services resulting from the use of this data set. CIAT also request reprints of any publications and notification of any redistributing efforts. For commercial access to the data, send requests to Andy Jarvis (a.jarvis@cgiar.org).
ACKNOWLEDGMENT AND CITATION
We kindly ask any users to cite this data in any published material produced using this data, and if possible link web pages to the CIAT-CSI SRTM website (http://srtm.csi.cgiar.org).
Create an object¶
from warsa.dem.srtm import SRTM3ARCSEC
srtm = SRTM3ARCSEC()
Create shapefile from tiles¶
The resulting shapefile has the file name of the corresponding tile as attribute.
srtm.create_layers()

Create a merged dem from shapefile¶
This procedure merges (mosaics) all SRTM 3 arcseconds tiles intersected by the given shapefile. They will also be downloaded if necessary. Example:
srtm.merge_by_layers('/media/win/PythonProjects/warsadev/data/BuziWatershedWGS84.shp',
output_raster='/media/win/tmp/srtm3_buzi.tif')
which is equivalent to:
tiles = srtm.get_tile_names_by_layers('/media/win/PythonProjects/warsadev/data/BuziWatershedWGS84.shp')
srtm.merge(tiles, output_raster='/media/win/tmp/srtm_buzi.tif')
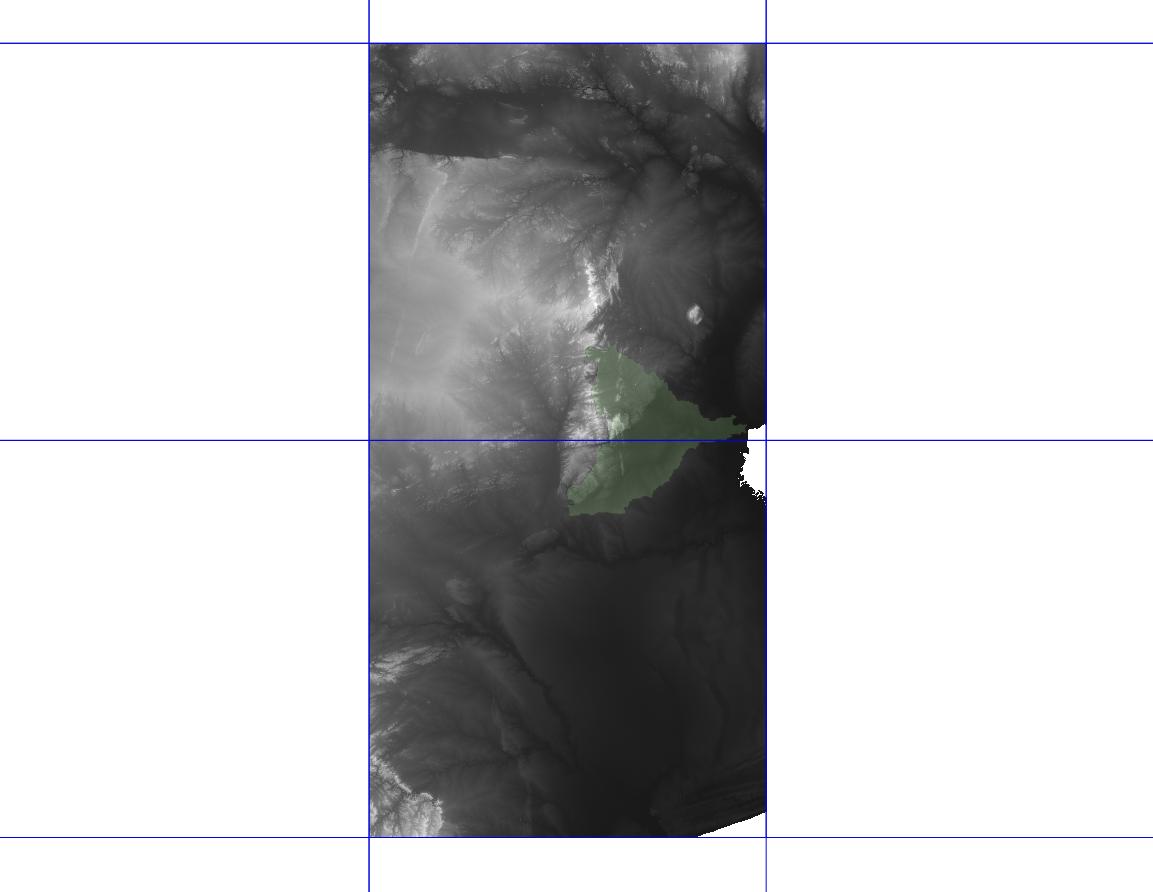
Download tiles¶
Tiles are downloaded in a directory srtm_1arcsec
, which is saved in the directory defined in the configuration file under dem
(see Configuration).
Downloading all tiles (it takes long):
srtm.download()
Output:
Downloading 7 SRTM 3 arc files
1: downloading srtm_01_02.zip... done in 1.97 seconds
2: downloading srtm_01_07.zip... done in 1.40 seconds
3: downloading srtm_01_12.zip... done in 2.84 seconds
4: downloading srtm_01_15.zip... done in 1.37 seconds
5: downloading srtm_01_16.zip... done in 4.49 seconds
6: downloading srtm_01_17.zip... done in 1.38 seconds
7: downloading srtm_01_18.zip... done in 1.42 seconds
Downloading tiles intersecting a shapefile:
srtm.download_by_layers('/media/win/PythonProjects/warsadev/data/BuziWatershedWGS84.shp')
Downloading tiles by name:
srtm.download_by_tile_names(['srtm_43_16.zip', 'srtm_43_17.zip'])
Output:
Downloading 2 SRTM 3 arcseconds files
1: downloading srtm_43_16.zip... done in 37.70 seconds
2: downloading srtm_43_17.zip... done in 20.96 seconds
Get tile names¶
print(srtm.get_tile_name_by_point(-67.5, -6.5))
print(srtm.get_tile_names_by_extent(-67.5, -64.5, -6.5, -6.3))
print(srtm.get_tile_names_by_layers('/media/win/PythonProjects/warsadev/data/BuziWatershedWGS84.shp'))
Output:
/media/roehrig/SARP/dem/srtm_3arcsec/srtm_23_14.zip
['/media/roehrig/SARP/dem/srtm_3arcsec/srtm_23_14.zip', '/media/roehrig/SARP/dem/srtm_3arcsec/srtm_24_14.zip']
['/media/roehrig/SARP/dem/srtm_3arcsec/srtm_43_16.zip', '/media/roehrig/SARP/dem/srtm_3arcsec/srtm_43_17.zip']